티스토리 뷰
unittest code를 작성하고 test code에서 module을 import할 때 너무나 많은 error가 발생했습니다. module을 찾을 수 없는 error와 attempted relative import with no known parent package
error 등의 error가 발생했습니다. 이러한 error가 발생한 원인을 정확하게 파악하려 합니다.
__name__
의 역할
python의 __name__
은 모듈이 저장되는 변수이며 import로 모듈을 가져왔을 때 모듈의 이름이 들어갑니다. 파이썬 인터프리터를 통해 파이썬파일을 직접실행할 경우에는 파이썬에서 알아서 그파일의 name은 __main__
이 됩니다. 파이썬 모듈을 import해서 사용할 경우에는 name은 원래 모듈 이름으로 설정됩니다. 그러므로 만약 해당 파일이 직접 실행시킬 때에만 실행되도록 설정하고 싶다면 if __name__ == '__main__':
로 실행합니다.
절대경로와 상대경로
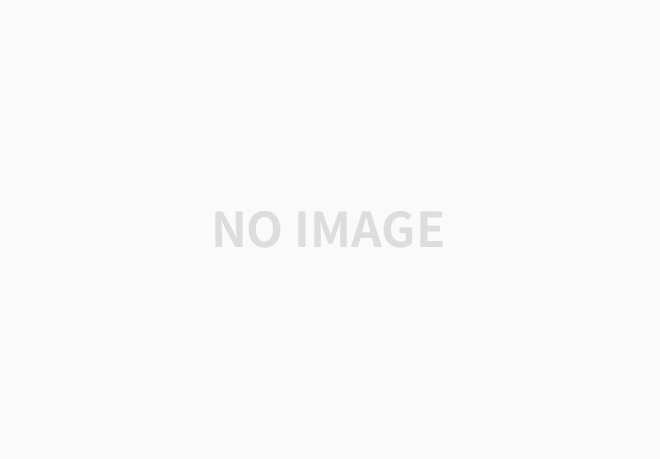
위와 같은 구조의 프로젝트가 있다고 한다고 합니다.
# add_two_numbers.py
from .lib.linked_list import LinkedList
상대경로는 내위치를 중심으로 표현한 경로를 말합니다. .
은 현재위치를 ..
은 상위 디렉터리를 나타냅니다.
# add_two_numbers.py
from lib.linked_list import LinkedList
절대경로는 절대경로는 변하지않는 고유경로입니다.
상대경로로 import하여 add_two_numbers.py
을 직접 실행하는 경우 attempted relative import with no known parent package
error가 발생합니다. 실행 후 상대경로를 통해 다른 모듈을 import 할때, 파이썬은 모듈의 이름 __name__
에 기반을 두고 현재모듈의 위치를 찾는다. __name__
이 직접 실행하는 경우 __main__
으로 변경되어 위치를 찾을 수가 없게 됩니다. 직접 실행하는 python file의 경우 항상 절대경로를 사용해야한다고 적혀있습니다.
Intra-package References: Note that relative imports are based on the name of the current module. Since the name of the main module is always "main", modules intended for use as the main module of a Python application must always use absolute imports.
sys.path
sys.path
는 디렉터리의 경로가 기록된 문자열 리스트입니다. 이 리스트에 경로를 추가하면 해당 경로에 있는 파이썬 파일을 import할 수 있습니다.
pip install <package>
명령을 실행하면 site-packages
folder에 package가 설치됩니다. sys.path
에 site-packages
folder의 path가 등록되어 있어 다운 받은 package를 절대경로로 import하여 사용할 수 있습니다.
sys.path
에는 기본적으로 몇 가지 경로가 미리 추가되어 있습니다. 직접 실행한 python file이 속한 folder의 위치가 추가되어 있습니다. 또한 위에서 언급한 site-packages
의 위치, python 인터프이터의 위치 등이 추가되어 있습니다.
unittest 사용하면서 경로 설정 문제점
실행 방법에 따라 다른 경로 설정
unittest의 테스트 케이스를 작성은 아래와 같습니다.
# tests/test.py
import unittest
class TestStringMethods(unittest.TestCase):
def test_upper(self):
self.assertEqual('foo'.upper(), 'FOO')
def test_isupper(self):
self.assertTrue('FOO'.isupper())
self.assertFalse('Foo'.isupper())
def test_split(self):
s = 'hello world'
self.assertEqual(s.split(), ['hello', 'world'])
# check that s.split fails when the separator is not a string
with self.assertRaises(TypeError):
s.split(2)
if __name__ == '__main__':
unittest.main()
이 테스트 케이스를 실행하는 방법은 python -m unittest test.py
로 실행합니다. 이와 같은 방법으로 실행하여 __name__
의 값을 확인하면 test
가 반환되는 것을 확인할 수 있습니다. __name__
에서 __main__
이 반환되지 않는 것으로 보아 직접실행되는 형태가 아닙니다. 직접실행하는 방법은 python test.py
로 실행하는 것입니다. python test.py
로 실행해야만 unittest.main()
이 실행됩니다.
python -m
의 실행은 모듈을 스크립트로 수행할 때 쓰는 옵션이라고 합니다. python -m unittest
로 실행하면 unittest module이 스크립트로 실행되고 test.py
는 unittest에 인자로 전달되어 import 형식으로 test.py
가 실행되는 것으로 보입니다.
python -m unittest test.py
로 실행하는 방법과 python test.py
로 실행하는 방법이 import하는 경로의 차이가 발생합니다. python test.py
로 직접 실행하는 경우 sys.path
의 경로에 tests
folder가 포함되지만 python -m unittest test.py
로 실해하는 경우 shell의 현재 위치가 sys.path
에 추가되어 있습니다. 따라서 경우 2가지 실행 방법에서 절대 경로를 설정한다면 다른 import 경로를 설정해야 합니다.
통합으로 test case를 실행할 때 다른 경로 설정
unittest로 test code를 작성할 때는 주로 하나의 파일만 실행합니다. test case를 작성을 완료하면 모든 test code를 한 번에 실행해야 합니다.
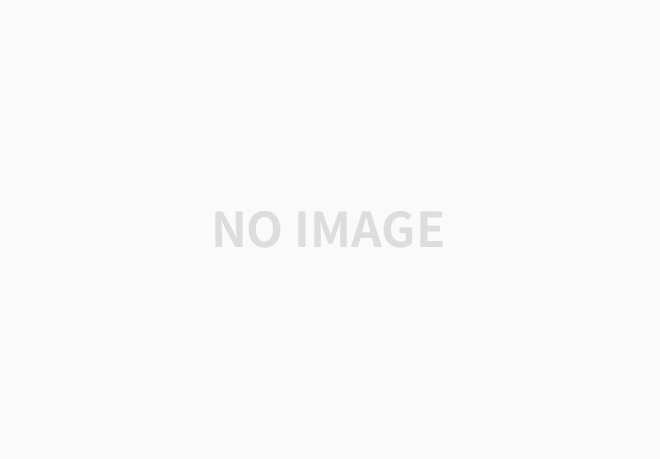
# test_attendace_service.py
import unittest
from unittest.mock import patch, MagicMock
from datetime import datetime
from flaskr.service import attendance_service
from flaskr.model import AttendanceModel, TopicModel, UserModel
.....
if __name__ == '__main__':
unittest.main()
절대경로로 module을 import하여 test_attendace_service.py
file 하나만 실행하는 경우 import 경로에 문제가 없이 동작하게 구성했습니다.
# all_tests.py
import glob
import unittest
import sys
import os
testdir = os.path.dirname(__file__)
sys.path.insert(0, os.path.abspath(
os.path.join(testdir, "../../des_api_lambda")))
if __name__ == '__main__':
loader = unittest.TestLoader()
start_dir = 'tests'
suite = loader.discover(start_dir)
runner = unittest.TextTestRunner()
runner.run(suite)
all_test.py
에서는 sys.path
에 project root folder의 위치를 추가하여 import 경로에 문제가 발생하지 않도록 구성했습니다.
Reference
'develop' 카테고리의 다른 글
Github Actions 관리하기(Organization secrets, Reusable workflows) (0) | 2022.06.29 |
---|---|
lambda@edge를 사용한 요청 header 처리(user-agent, accept-language) (0) | 2022.01.05 |
DAS / NAS / SAN (0) | 2021.10.29 |
3 계층 architecture (0) | 2021.10.29 |
AWS KMS (0) | 2021.10.17 |
- Total
- Today
- Yesterday
- AWS community day seoul
- graphql
- lambda@edge
- conventional commit
- commit message
- Cognito
- Airflow
- NLP
- Lifecycle
- Neptune
- nltk
- Prisma
- mognodb
- sementic version
- typescript
- Clickjacking
- nginx
- inversify
- slowquery
- Python
- Github Actions
- mongoDB
- Cloudfront
- Terraform
- Elasticsearch
- Develop
- pagination
- shorten
- JavaScript
- aws
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |